27 Nov 2018
|
Javascript
JQuery
개인적으로 자주 쓸 것 같아서 보관용으로 포스팅한다.
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Timestamp Converter</title>
<script
src="https://code.jquery.com/jquery-1.12.4.js"
integrity="sha256-Qw82+bXyGq6MydymqBxNPYTaUXXq7c8v3CwiYwLLNXU="
crossorigin="anonymous"></script>
<body>
<textarea id="timestamp_list" style="height: 200px"></textarea>
<button id="time_btn">변환</button>
<div id="conv_list">
</div>
<script>
$("#time_btn").on("click", function() {
var $timestamp_list_val = $("#timestamp_list").val();
var lists = $timestamp_list_val.split("\n");
var conv_lists = [];
$.each(lists, function(ind, val) {
var convDate = new Date(Number(val));
conv_lists.push(convDate.toLocaleString());
});
$("#conv_list").html(conv_lists.join("<br/>"));
});
</script>
</body>
</html>
27 Nov 2018
|
Spring
Java
언제 사용하면 좋을까?
- 요청이 긴 경우
- 로그 처리
- 푸시 처리
Async 기능 켜기 Enable Async Support
자바 설정(Java configuration) 관련 클래스에 @EnableAsync 를 추가해주기만 하면 된다.
@Configuration
@EnableAsync
public class SpringAsyncConfig { ... }
Config
@EnableAsync만 추가하면 기본적인 설정은 끝이다.
하지만, 기본값인 SimpleAsyncTaskExecutor 클래스는 매번 Thread를 만들어내는 객체이기 때문에 Thread Pool이 아니다.
Thread Pool을 설정해기 위해 AsyncConfigurerSupport를 상속받아 재구현하자.
@Configuration
@EnableAsync
public class SpringAsyncConfig extends AsyncConfigurerSupport {
@Override
public Executor getAsyncExecutor() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(2);
executor.setMaxPoolSize(10);
executor.setQueueCapacity(500);
executor.setThreadNamePrefix("heowc-async-");
executor.initialize();
return executor;
}
}
선언 후 호출하기.
어노테이션 선언
- 비동기 작업을 하기 위한 메소드에 @Async를 추가하면 된다.
만약 callback이 필요하다면, Future 클래스 등의 객체로 감싸서 반환하면 된다.
@Component
@Slf4j
public class MessageSender {
/**
* @param to
* @param subject
* @param text
*/
@Async
public void sendSimpleMessage(String to, String subject, String text) {
sendSimpleMessageSync(to, subject, text);
}
public void sendSimpleMessageSync(String to, String subject, String text) {
.....
}
}
호출은 아래와 같이 하면 된다.
@Autowired
private MessageSender messageSender;
public void sendEmail(String originCd, String subject, String to, String companyCd, String productCd, String reservCd, String templateID, String dailCode, Map valueMap){
...
messageSender.sendSimpleMessage(to, subject, html);
...
}
참고:
- https://heowc.github.io/2018/02/10/spring-boot-async/
27 Nov 2018
|
Git
Intellij
로컬에만 커밋 되어있고 push 처리가 되지 않았을 경우에 할 수 있는 2가지 방법.
- IntelliJ 2017.1 이상 => 로그로 이동하여 + 단어를 오른쪽 클릭 후 F2 키를 눌러서 커밋메세지를 변경할 수 있다.
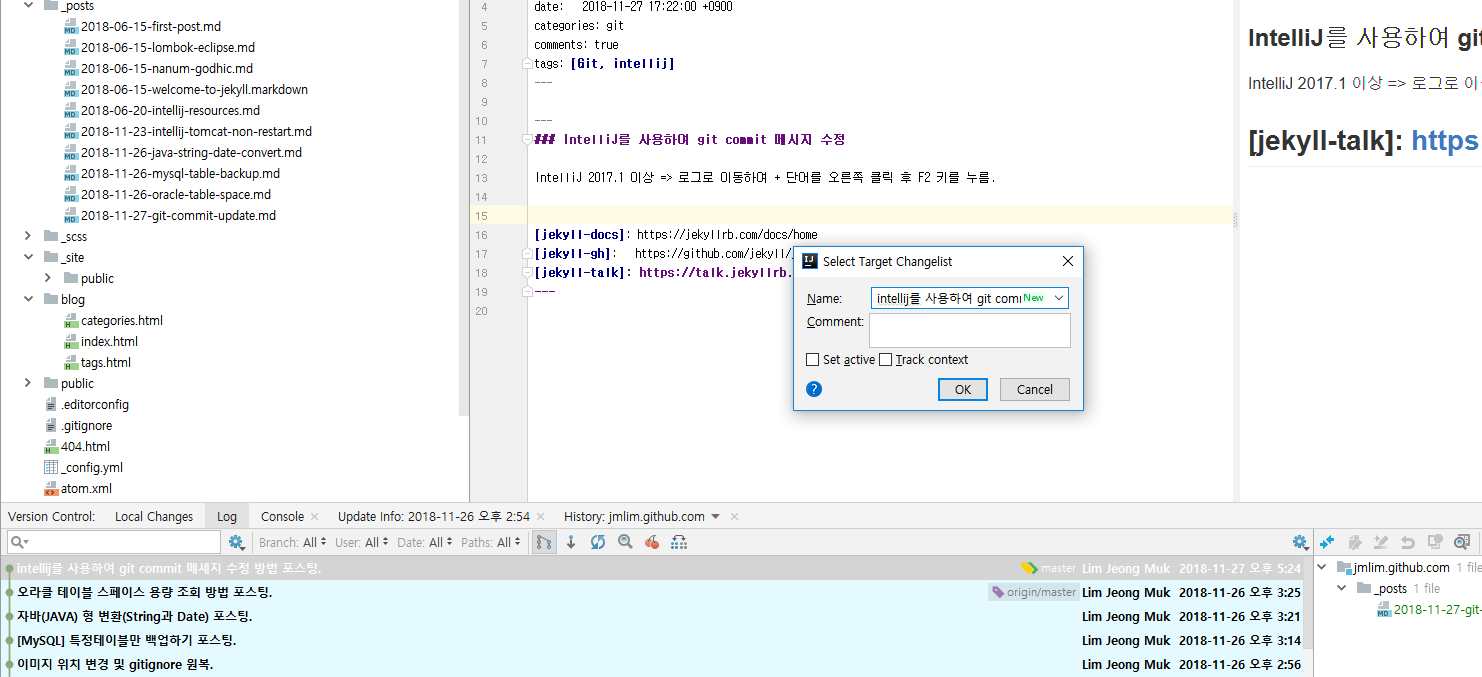
- jetBrains Go to View -> Version Control -> Log 탭 선택 후 오른쪽 마우스를 클릭하고 undo commit 을 선택하면 다시 커밋할 수 있다.
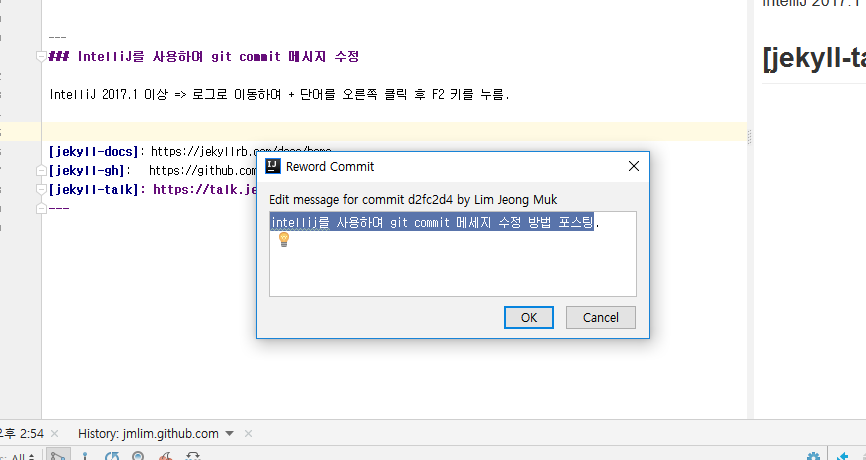